Flow Sensors
Flow sensors are sensors that measure the flow rate of a fluid or gas. They are used in a wide range of applications, from measuring the flow of water in a pipe to monitoring the flow of air in an engine.
There are many different physical principles that can be used to measure flow rate. Some common types of flow sensors are:
- Hall effect based flow sensors: These sensors use a impeller with a magnet and a hall effect sensor to detect a rotation of the impeller.
- Ultrasonic flow sensors:
- Thermal mass flow sensors: They combine a heater element and a temperature sensor, and measure the amount of energy absorbed by the fluid as it passes over the heater.
- Differential pressure flow sensors: These utilize the Bernoulli principle to measure the pressure drop across an obstruction as the fluid flows through it.
Hall Effect Flow Sensors
MCU Drivers
A microcontroller driver for a hall effect sensor is very easy to write, given the simple digital pulse output from the sensor.
Below is some C pseudo code for a hall effect flow sensor driver:
// Access to this has to be atomic. See the notes below.volatile uint32_t pulseCount = 0;
uint64_t lastMeasurementTime_us = 0;
int main() { // Setup the interrupt handler for the hall effect sensor configure_interrupt(SENSOR_GPIO_PIN, RISING_EDGE, sensor_isr);
while(1) { uint64_t currentTime_us = get_time_us();
uint64_t timeSinceLastMeasurement_us = currentTime_us - lastMeasurementTime_us;
// Assuming there is an atomic_clear() function which // clears the atomic variable and returns the previous value uint32_t pulseCountCopy = atomic_clear(&pulseCount);
// F = 6.6 * Q where F is the frequency of the hall effect signal, Q is the flow rate in L/min // Q = F / 6.6 // Q = 1 / 6.6 * t where t is the period, t = 1 /F // Since we have measured N pulses in t_total, t_period = t_total / N, // Thus Q = 1 / 6.6 * (t_total / N) // Q = N / 6.6 * t_total double floatRate_Lpmin = (double)pulseCount / (6.6 * (double)timeSinceLastMeasurement_us / 1000000.0); printf("Flow rate: %.02f L/min\n", floatRate_Lpmin);
lastMeasurementTime_us = currentTime_us; sleep_ms(1000); }}
/** * This gets called on the rising edge of the hall effect signal */void sensor_isr() { atomic_inc(&pulseCount);}
If you do not have any atomic variables available, you have two other options:
- Disable interrupts when reading/clearing the pulse count from
main()
. - Use a mutex and lock it when reading/clearing the pulse count from
main()
(this assumes you are using an RTOS).
Be aware that myVar++
is generally not atomic, even if reading and writing are atomic since ++
(incrementing) involves a read and a write.
I used doubles since I was running on a rather fast microcontroller with FPU support. If using doubles gives you performance issues, you could either use floats or fixed point arithmetic.
I also configured the ISR to be called only on the rising edge of the hall effect signal. This was because triggering on both edges doesn’t really give you any more information, and I didn’t want to make the assumption that the hall effect signal outputted a 50% duty cycle square wave at all speeds (if it strayed far from 50% and you were using data from both edges, you could get some poor flow rate measurements at slower speeds).
Examples
YF-Bx
YF-Bx is a family of hall effect based flow sensors mounted in a small piece of 1” brass tubing. It can measure flow rates between 1 and 25 L/min. The YF-Bx family have a working voltage of 5V to 15V(DC) and the G1&x family have a working voltage of 5V to 24V(DC).
There are three wires:
- Red: +VCC
- Black: GND
- Yellow: Signal
The yellow signal wire outputs a digital signal which transitions between high and low a fixed number of times per revolution of the impeller (using the hall effect sensor). It has a duty cycle of 50%+-10%.
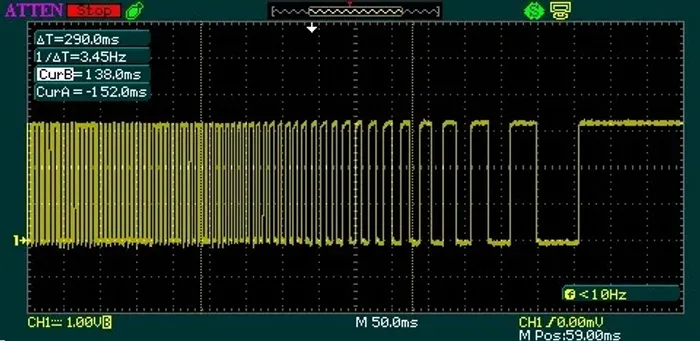
The rate is typically expressed as a frequency. For example, the YF-B6 datasheet describes a frequency of F=6.6*Q(Q=L/MIN)
.1 F is the measured frequency of the hall effect signal, and Q is the flow rate in L/min. In a microcontroller, you would typically setup a GPIO interrupt on the sensor pin, and then measure the time between pulses. To calculate the flow rate, we would need to re-arrange the equation. Let’s also substitute for the period () since that’s what we’ll actually measure.
where:
is the frequency of the hall effect signal in Hz
is the flow rate in L/min
is the time between pulses in seconds
As you normally want to accumulate a number of pulses over a fixed period of time (e.g. 1s), and then calculate the flow rate, you need to modify the equation slightly. Let’s call the number of pulses and the total time period these were measured over .
where:
is the number of pulses measured over a fixed period of time
is the total time period over which the pulses are measured
So therefore:
The differences between the YF-Bx and G1&x families are:
- YF-Bx: Have a brass tube and a working voltage of 5V to 15V(DC).
- G1&x: Have a plastic tube and a working voltage of 5V to 24V(DC).
This is a placeholder for the reference: tbl-yf-bx-specs shows the core specifications for the flow sensors in the YF-Bx and G1&x families. For the frequency equation, is the frequency of the hall effect signal in Hz, is the flow rate in L/min.
Type | Dimensions (DN) | Working Voltage | Flow Rate Range | Frequency Eq. | Length | Male & Female | Length of Thread | Material |
---|---|---|---|---|---|---|---|---|
YF-B1 | DN15 | 5V to 15V(DC) | 1 to 25L/min | - | 44mm | Double Male | 10mm | Copper |
YF-B2 | DN15 | 5V to 15V(DC) | 1 to 25L/min | - | 50mm | Male in Female out | 10mm | Copper |
YF-B3 | DN15 | 5V to 15V(DC) | 1 to 25L/min | - | 66mm | Double Male | 18mm | Copper |
YF-B4 | DN15 | 5V to 15V(DC) | 1 to 25L/min | - | 66mm | Male in Female out | 10mm | Copper |
YF-B5 | DN20 | 5V to 15V(DC) | 1 to 30L/min | F=6.6*Q | 50mm | Double Male | 10mm | Copper |
YF-B6 | DN20 | 5V to 15V(DC) | 1 to 30L/min | F=6.6*Q | 60mm | Double Male | 11mm | Copper |
YF-B7 | DN15 | 5V to 15V(DC) | 1 to 25L/min | - | 66mm | Double Male | 10mm | Copper |
G1&2 | DN15 | 5V to 24V(DC) | 1 to 30L/min | - | - | Double Male | - | Plastic |
G3&4 | DN20 | 5V to 24V(DC) | 1 to 60L/min | - | - | Double Male | - | Plastic |
G1&8 | - | 5V to 24V(DC) | 0.3 to 6L/min | - | - | - | - | Plastic |
M11*1.25 | - | 5V to 24V(DC) | 0.3 to 6L/min | - | - | - | - | Plastic |
There is also the YF-S201 flow sensor which seems related.
Tiny Flow Sensors
Tiny flow sensors exist in chip-size packages. These are in a group of products called MEMS (micro-electro-mechanical system) devices.
Sensirion makes small 10x10mm liquid flow sensors (the LPP10 and LPG10). They are based on thermal MEMs elements. They can measure between 0 and 1000uL/min (1mL/min), with a resolution that can be as low as 0.5nL/min. It has a response time 40ms/sample. It is compatible with H20, methanol, ethanol, and blood.
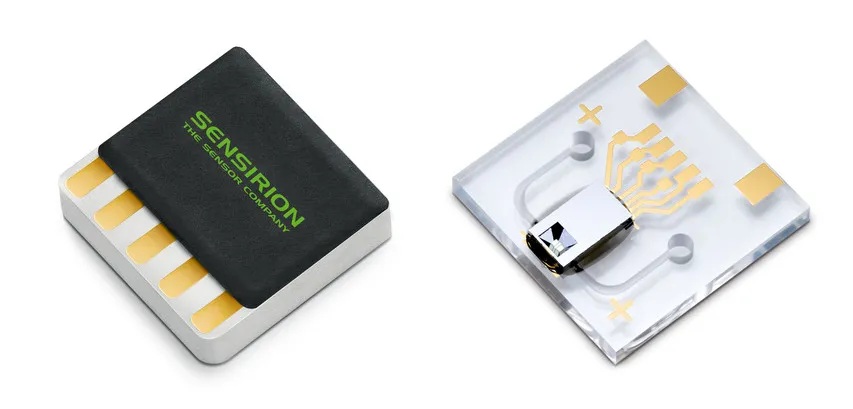
Further Reading
See Hall effect sensors for more information on the hall effect principle and how Hall effect sensors are built.
See Encoders for more information on how encoders work and how they can be connected to a microcontroller.
Footnotes
-
Seeed Studio. Water Flow Sensor YF-B6 [datasheet]. Retrieved 2025-02-25, from https://media.digikey.com/pdf/data%20sheets/seeed%20technology/114991176_web.pdf. ↩
-
Seeed Studio. Home / The Devices / Data Collection Systems / Energy Management Units / Water Flow Sensor YF-B6 [product page]. Retrieved 2025-02-24 from https://www.seeedstudio.com/Water-Flow-Sensor-YF-B6-p-2883.html. ↩